Public Fidelity
Member
Thread Starter
- Joined
- May 6, 2024
- Messages
- 6
Hey everyone!
Just wanted to share a quick project I recently worked on: an automated loudspeaker measurement turntable controlled directly from REW (Room EQ Wizard).
I built this turntable to streamline my loudspeaker measurements and make the process more efficient. With REW's API-control (Beta release), I can precisely rotate the turntable to capture data at different angles, allowing for spinorama measurements without the need for manual adjustments. The setup has been a game-changer for me, saving time and ensuring accuracy in my measurements.
Here are some pictures with a small explainer:
The table (made from the Audiomatica Medusa project files)
Table in action
I wrote a custom controller that uses the REW-API (in beta release)
This is log from controller:
It uses the measurement 'Increment' number as rotational distance input for the turntable.
The 'Repeated measurement' feature in REW allows for automated repeat.
Example of 0 - 80 degrees measurement sequence (gated)
Happy to answer any questions or share more details if anyone's interested.
Just wanted to share a quick project I recently worked on: an automated loudspeaker measurement turntable controlled directly from REW (Room EQ Wizard).
I built this turntable to streamline my loudspeaker measurements and make the process more efficient. With REW's API-control (Beta release), I can precisely rotate the turntable to capture data at different angles, allowing for spinorama measurements without the need for manual adjustments. The setup has been a game-changer for me, saving time and ensuring accuracy in my measurements.
Here are some pictures with a small explainer:
The table (made from the Audiomatica Medusa project files)
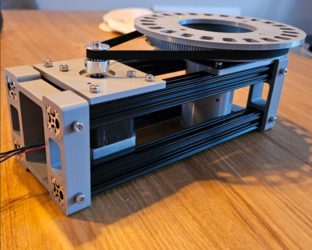
Table in action
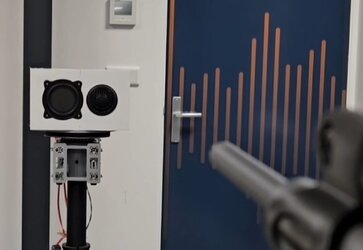
I wrote a custom controller that uses the REW-API (in beta release)
This is log from controller:
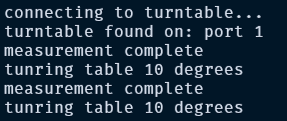
It uses the measurement 'Increment' number as rotational distance input for the turntable.
The 'Repeated measurement' feature in REW allows for automated repeat.
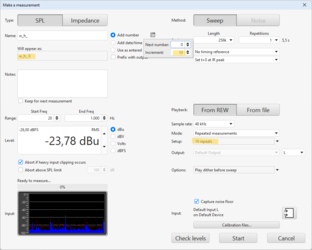
Example of 0 - 80 degrees measurement sequence (gated)
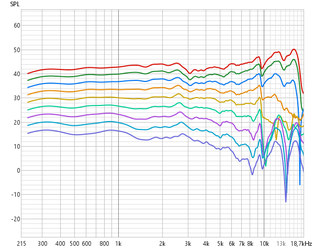
Happy to answer any questions or share more details if anyone's interested.
Last edited: